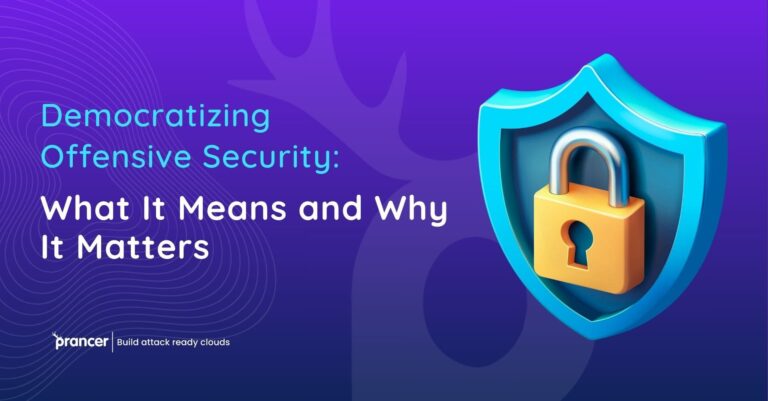
Mass Assignment refers to the risk of insecurely handling user input in APIs, which can allow attackers to modify or manipulate data in unintended ways. This can occur when APIs do not properly validate or sanitize user input, or when APIs allow direct assignment of user input to object properties without proper authorization or validation.
Some common risks associated with Mass Assignments include:
Attack scenarios for cloud applications may include:
A vulnerable sample of code in Go lang might look like this:
type User struct {
ID int `json:"id"`
Email string `json:"email"`
Password string `json:"password"`
FirstName string `json:"first_name"`
LastName string `json:"last_name"`
Role string `json:"role"`
}
func updateUser(w http.ResponseWriter, r *http.Request) {
// Get the user's ID from the request
userID := r.Header.Get("X-User-ID")
// Get the updated user data from the request body
var user User
err := json.NewDecoder(r.Body).Decode(&user)
if err != nil {
http.Error(w, "Error decoding request body", http.StatusBadRequest)
return
}
// Update the user in the database
err = database.UpdateUser(userID, user)
if err != nil {
http.Error(w, "Error updating user", http.StatusInternalServerError)
return
}
// Return a success message to the user
json.NewEncoder(w).Encode("User updated successfully")
}
In this example, the API call allows a user to update their own data in a database. However, the API directly assigns the user input from the request body to the properties of a User struct without any validation or authorization checks. An attacker could exploit this vulnerability by intercepting the API call and modifying the request body to update the user’s data in unintended ways, such as changing the user’s role or password.
A sample attack payload using the curl command might look like this:
curl -H "X-User-ID: attacker_user_id" -d '{"email":"attacker@example.com",
"password":"attacker_password","first_name":"Attacker","last_name":"Attacker",
"role":"admin"}' -X PUT http://api.example.com/updateuser
In this example, the attacker is using curl to send a PUT request to the API with a modified user ID in the request header and a modified request body that includes a new email, password, and role for the user. If the API is vulnerable to Mass Assignment, the attacker may be able to update the user’s data in unintended ways.
Mass Assignment can be mapped to the Tactic: Privilege Escalation and the Techniques: Exploitation of Uncontrolled Linkage to a Third-party
Our in-depth whitepaper provides valuable insights into how Prancer Security’s cutting-edge solution mitigates critical risks such as unauthorized access and data breaches, while adhering to the highest security standards.
Don’t leave your API security to chance – download our comprehensive whitepaper now and discover how Prancer Security can safeguard your organization from potential threats!